We are like the tip of an iceberg.
There may be a lot more to each of us, but there’s only so much that we choose to show. And, when we do, i.e., when we post something for others to see, we like to (obsessively) track how many (and who!) saw it. After all, even icebergs like a little attention…
Jokes aside, who doesn’t like data?
I track page view counts using Google Analytics (GA) for each post on this WordPress blog running Twenty Twenty theme, and show it on the page (as shown in the picture) using the Google Analytics Reporting API v4.
If you like the count, here’s how you can add it to your WP blog.
If you haven’t already, setup a GA account, install Site Kit plugin, and connect it with that account. There are plenty of tutorials available for all of that.
Once your page views start getting counted on GA, head over to Query Explorer to play around with the dataset and look for page views. In particular:
- Set
start-date
to the date you implemented GA - Set
end-date
totoday
- Set
metrics
toga:pageviews
- Set
dimensions
toga:pagepath
This should return a table with all the pages, as dimension, and their page views, as metric. Once that works, you know what the end result will look like.
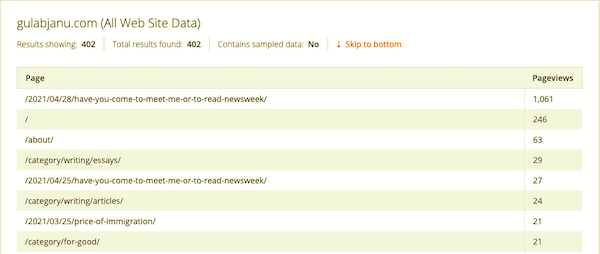
If you want to filter for one particular page, set filters
to ga:pagePath==/your/page/permalink/minus/domain
. This is what we will achieve programatically in the next step.
Now, let’s setup access to the reporting API.
Do everything under ‘Step 1’ here to create a project, enable the API, and create the access credentials.
Next, download the latest version of Google APIs Client Library for PHP, extract the zip, and, using FTP or File Transfer, upload it in /wp-content/themes/twentytwenty/inc/
. Now, relax; it will takes a few minutes.
In step 3, you had generated a JSON key. Upload it in the same folder as step 4.
We will now add functions to the code.
There’s a file in that same folder called template-tag.php
; open it in Theme Editor or download it and open it in a code editor.
Look for the “post meta” section because that’s where I added the view count. It should be around line 224.
Add this line to include the client library you uploaded:
require_once DIR . '/google-api-php-client--PHP8.0/vendor/autoload.php';
Next, let’s add the first function that will initialize the service account using the JSON key you uploaded. Make sure you replace “your-security-token” with the name of your file.
/**
* Initializes an Analytics Reporting API V4 service object.
*
* @return An authorized Analytics Reporting API V4 service object.
*/
function initializeAnalytics()
{
// Use the developers console and download your service account
// credentials in JSON format. Place them in this directory or
// change the key file location if necessary.
$KEY_FILE_LOCATION = __DIR__ . '/your-security-token.json';
// Create and configure a new client object.
$client = new Google_Client();
$client->setApplicationName("Google Analytics API Reporting");
$client->setAuthConfig($KEY_FILE_LOCATION);
$client->setScopes(['https://www.googleapis.com/auth/analytics.readonly']);
$analytics = new Google_Service_AnalyticsReporting($client);
return $analytics;
}
With that, we can add the next function that will query the API to get page views. Please replace “ga:999999999” in $VIEW_ID
with your GA Profile ID. Also, set start-date
to when you implemented GA. Finally, as I said above, the query will automatically filter the results to views for the current page using $_SERVER['REQUEST_URI']
.
/**
* Queries the Analytics Reporting API V4.
*
* @param service An authorized Analytics Reporting API V4 service object.
* @return The Analytics Reporting API V4 response.
*/
function getReport($analytics) {
// Replace with your view ID, for example XXXX.
$VIEW_ID = "ga:999999999";
// Create the DateRange object.
$dateRange = new Google_Service_AnalyticsReporting_DateRange();
$dateRange->setStartDate("2020-01-01");
$dateRange->setEndDate("today");
// Create the Metrics object.
$pageViews = new Google_Service_AnalyticsReporting_Metric();
$pageViews->setExpression("ga:pageviews");
$pageViews->setAlias("page views");
//Create the Dimensions object.
$pagePath = new Google_Service_AnalyticsReporting_Dimension();
$pagePath->setName("ga:pagepath");
$dimensionFilter = new Google_Service_AnalyticsReporting_DimensionFilter();
$dimensionFilter->setDimensionName("ga:pagepath");
$dimensionFilter->setOperator("EXACT");
$dimensionFilter->setExpressions(array($_SERVER['REQUEST_URI']));
// Create the DimensionFilterClauses
$dimensionFilterClause = new Google_Service_AnalyticsReporting_DimensionFilterClause();
$dimensionFilterClause->setFilters(array($dimensionFilter));
// Create the ReportRequest object.
$request = new Google_Service_AnalyticsReporting_ReportRequest();
$request->setViewId($VIEW_ID);
$request->setDateRanges($dateRange);
$request->setDimensions(array($pagePath));
$request->setDimensionFilterClauses(array($dimensionFilterClause));
$request->setMetrics(array($pageViews));
$body = new Google_Service_AnalyticsReporting_GetReportsRequest();
$body->setReportRequests( array( $request) );
return $analytics->reports->batchGet( $body );
}
Finally, we add the function that will parse the results of the query and return what we’re after.
/**
* Parses and prints the Analytics Reporting API V4 response.
*
* @param An Analytics Reporting API V4 response.
*/
function printResults($reports) {
$pageViews = '0';
if (count($reports) > 0) {
$report = $reports[0];
$rows = $report->getData()->getRows();
if (count($rows) > 0) {
$row = $rows[0];
$metrics = $row->getMetrics();
if (count($metrics) > 0) {
$values = $metrics[0]->getValues();
if (count($values) > 0) {
$pageViews = $values[0];
}
}
}
}
return $pageViews;
}
With that, we are done with the setup, and can now call the functions to initialize the service account, query the API, and parse the results.
All that’s left to do is to call those functions and display the results in the post-meta
function.
Look for function twentytwenty_get_post_meta( ... )
.
Scroll down to apply_filters
and in the array, along with author
, post-date
, comments
, and sticky
, and add "views"
.
$post_meta = apply_filters(
'twentytwenty_post_meta_location_single_top',
array(
'author',
'post-date',
'comments',
'sticky',
'views',
)
);
Finally, scroll further down, past the Sticky
section, and paste the following code to add a section for Views
.
// Views.
if ( in_array( 'views', $post_meta, true ) && !is_archive() ) {
$has_meta = true; ?>
<li class="post-sticky meta-wrapper">
<span class="meta-icon">
<?php twentytwenty_the_theme_svg( 'search' ); ?>
</span>
<span class="meta-text">
<?php $analytics = initializeAnalytics(); $response = getReport($analytics); $count = printResults($response); printf(
/* translators: %s: View count. */ __( 'Views: %s', 'twentytwenty' ),$count);
?>
</span>
</li>
<?php
}
There are a few things going on here.
$analytics = initializeAnalytics();
initializes the service account.$response = getReport($analytics);
queries the API, passing the URL of the current page as a parameter.$count = printResults($response);
returns the page views count, if any.printf(__( 'Views: %s', 'twentytwenty' ),$count);
prints the count.twentytwenty_the_theme_svg( 'search' );
displays the search symbol as the count icon (because I needed one).- Finally, I added
&& !is_archive()
in the if-condition at the top to prevent the count from showing in the article summary on a category page, or an archive page. The count would be wrong in that instance because it would be showing the count for that category or archive page, and not the actual post.
That’s about it. On each blog post, you should now see a good-looking post views count below the title.
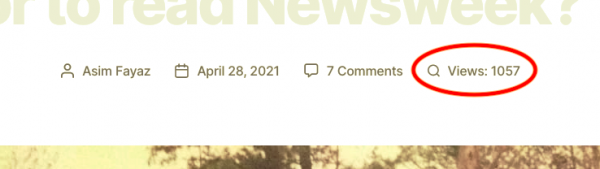
I have to admit; it took me a few hours to pull this off because I didn’t find any clear guidance in the API documentation, or on Stack Overflow. The fact that I hadn’t tinkered in PHP in years didn’t help either. Regardless, here we are. Hopefully it wouldn’t take you as long.
P.s. You’ll probably need to enable debugging (in wp-config.php
) so you can see where you messed up and fix it easily.